How you can use Directus GraphQL on your website?
GraphQL is a very powerful API for retrieving your data from Directus. In this article, I'll show you how you can query, create, update and delete records.
To use GraphQL on your website you will first need an Authentication Token from Directus. Then you can use your favourite server-side scripting language to the perform queries.
But, how does this work?
The idea behind using an API with your website is to dynamically pull data from Directus and display it to the user. This can be page content, user information, products, images etc. The website essentially becomes a shell.
As and example, lets say the homepage is opened, you can have a query to fetch the website's main menu, then a query to find the homepage banner. Then several queries to fetch featured content. After each query is made, you will need to process the data into HTML. This can be from template library or coded into a page template.
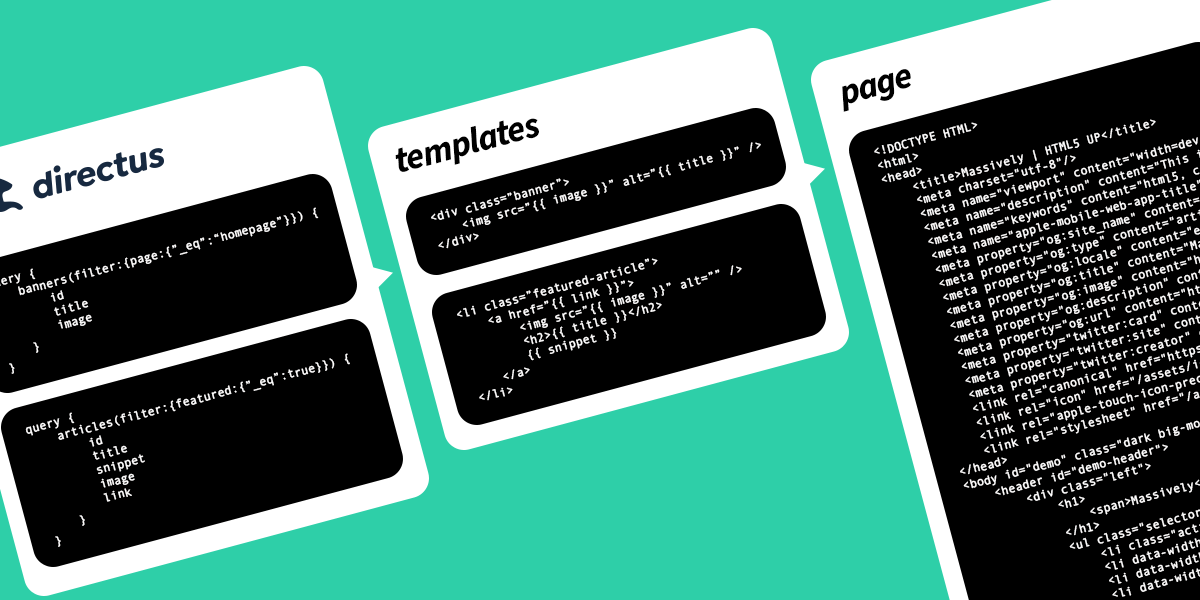
Alternatively, you can use the javascript SDK from Directus.
For this article, I use a free application called Postman which supports GraphQL and use it to test my API queries and visualise the responses. Let's dive into the first segment of GraphQL - Authentication.
Authentication
In Directus, you will need an API user and a dedicated role for setting permissions for the API. The user will have a token defined in the User Management portal. This token is used in the Bearer Token authentication header.
You can test the API using the free tool Postman. Create a new Collection and open the Authorization tab and paste you token into the Token field at the bottom. Make sure the Type is Bearer Token.
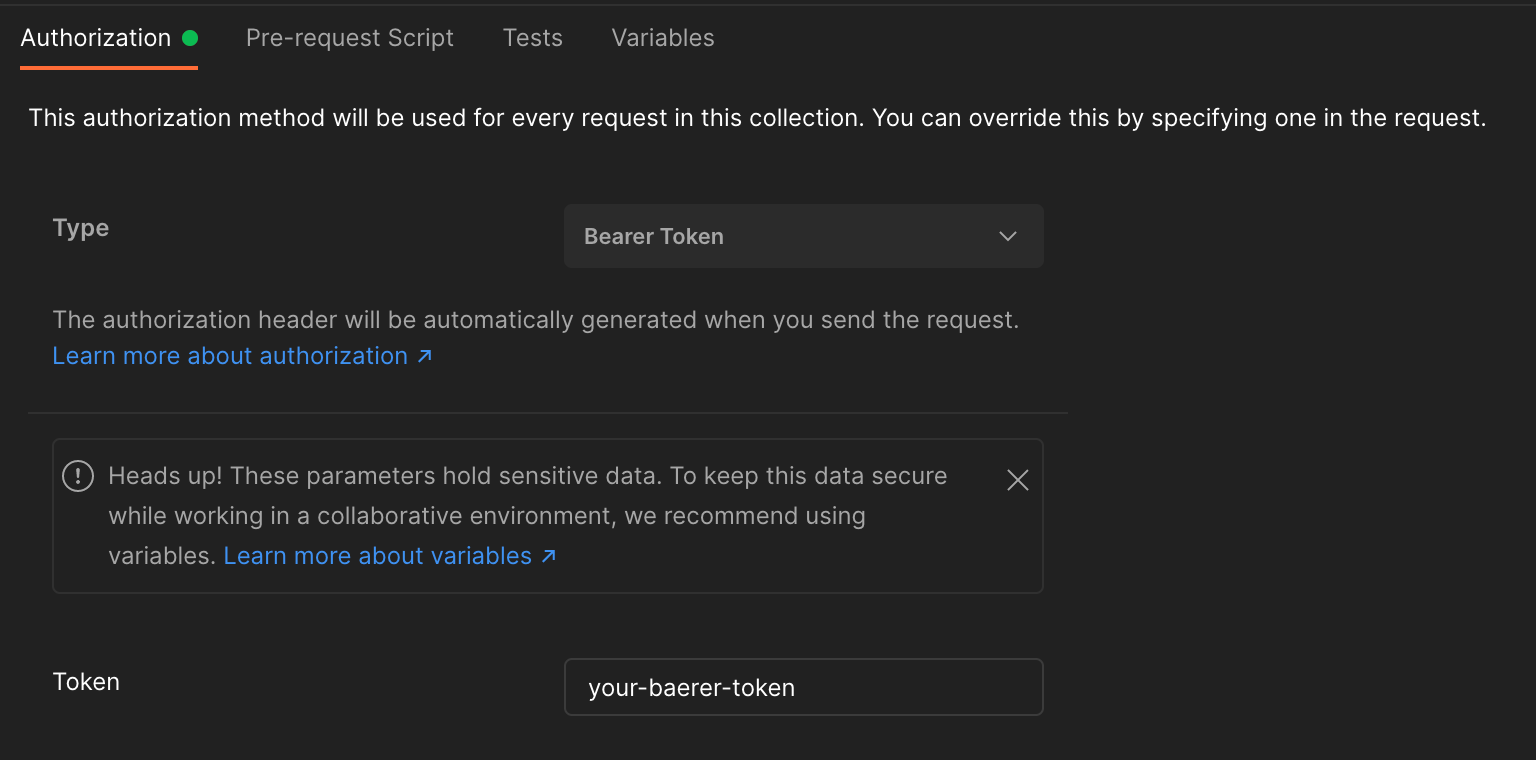
For you application or website, you need to add the token to the authorization header. I'm writing these commands below in raw curl which can be easily translated into your chosen language.
curl -H "Authorization: Bearer your-bearer-token"
Now that you have your token in Postman and your script, we can try running seom queries.
Query the API
GraphQL just has the one endpoint for all queries.
https://directus.example.com/graphql
To specify what collection and fields to query, you must POST some information to the address above. This is a unique format also called GraphQL
query {
articles {
id
title
author {
first_name
}
}
}
In Postman, create a new Request and choose POST as the method. The URL is the GraphQL endpoint as mentioned above. Lastly, click on the Body tab and choose GraphQL from the choices. Paste the GraphQL query into the Query box as seen below:
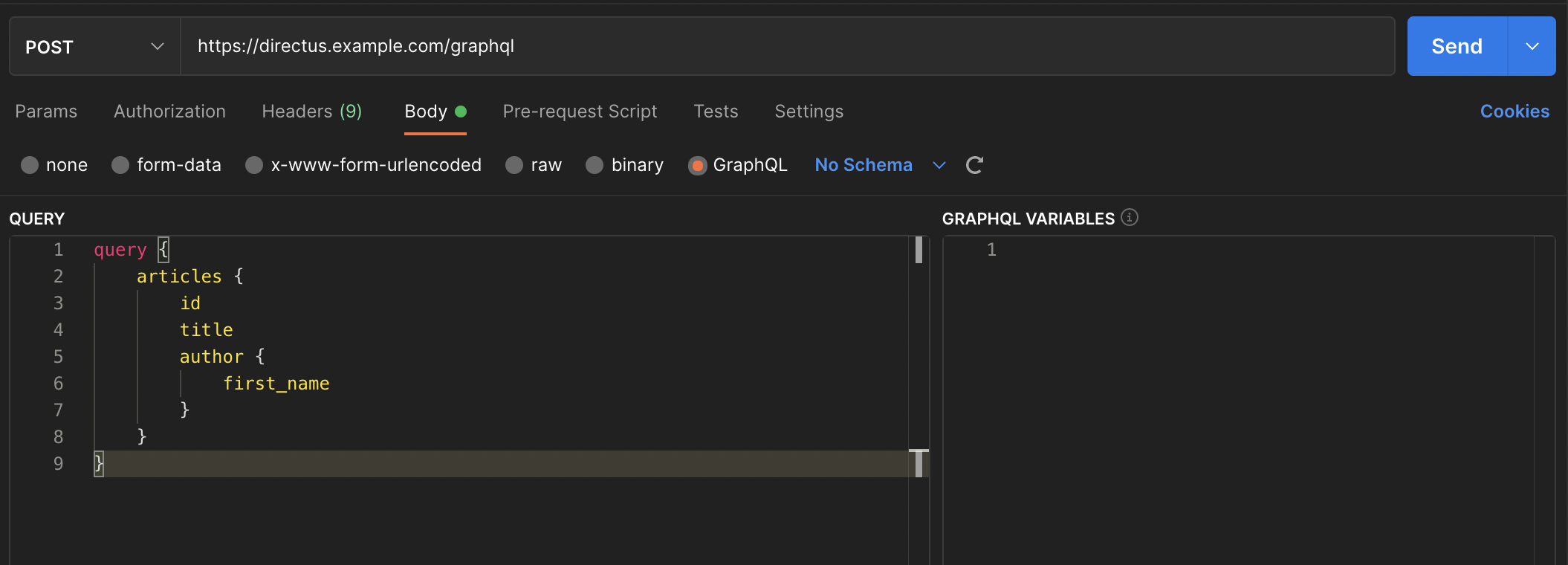
Once you click Send, you will receive a JSON response.
{
data: [
{
"id": 1,
"title": "Hello, world!",
"author": {
"first_name": "Robert"
}
}
]
}
The query in CURL is a little different. You will need to collapse your GraphQL into one line, then wrap it in a JSON array:
{"query": "GraphQL query goes here"}
So the query will look like this:
curl -H "Authorization: Bearer your-bearer-token" -X POST -d '{"query": "query { articles { id title author { first_name }}}"}' https://directus.example.com/graphql
But how do I query a specific record?
To query a specific record, you'll need to change your GraphQL query to:
query {
articles_by_id(id: 15) {
id
title
author {
first_name
}
}
}
The JSON response is slightly different, where the data value is no longer a list.
{
data: {
"id": 15,
"title": "More Information about this",
"author": {
"first_name": "Sarah"
}
}
}
Using what we know about CURL from the previous query, we'll wrap the GraphQL query in JSON again:
curl -H "Authorization: Bearer your-bearer-token" -X POST -d '{"query": "query { articles_by_id(id: 15) { id title author { first_name }}}"}' https://directus.example.com/graphql
At this stage you can the majority of you websites or application, but what if you need to create records into Directus.
Creating a New Record
This is where GraphQL really shines. We know how to make a query, now we'll use the same approach to create a new record in the articles table.
To create a new record, you must POST the following query:
mutation {
create_articles_item(data: { title: "Hello world!", body: "This is our first article" }) {
id
title
}
}
Notice the query has changed to "mutation" and the content is supplied as JSON inside the brackets. Lastly, the scope { id title } is requesting those fields be returned in the response. Here is an example of the response:
{
data: {
"id": 16,
"title": "Hello world!"
}
}
Remember the GraphQL must be wrapped in JSON. So the CURL command looks like this.
curl -H "Authorization: Bearer your-bearer-token" -X POST -d '{"query": "mutation { create_articles_item(data: {title:"Hello world!", body:"This is our first article"}) { id title }}"}' https://directus.example.com/graphql
Now that we can create records, it will be useful to update records too.
Update a Record
This is very similar to the creation method. To update a record, you must POST the following query:
mutation {
update_articles_item(id: 16, data: { title: "Updated Title!" }) {
id
title
}
}
And the response includes the requested id and title:
{
data: {
"id": 16,
"title": "Updated Title!"
}
}
Remember the GraphQL must be wrapped in JSON. So the CURL command looks like this.
curl -H "Authorization: Bearer your-bearer-token" -X POST -d '{"query": "mutation { update_articles_item(id: 16, data: {title:"Updated Title!"}) { id title }}"}' https://directus.example.com/graphql
Lastly, it's useful to know how to delete a record.
Delete a Record
It's best to avoid delete data from a database. Directus has a useful archive status that essentially soft-deletes the record using the update query above. Otherwise, if your need the permenantly delete a record, use the query below.
Again, this is very similar to the creation and update method. To delete a record, you must POST the following query:
mutation {
delete_articles_item(id: 16) {
id
}
}
The response will be empty if successful. You'll know if something goes wrong when you get a JSON response.
Remember the GraphQL must be wrapped in JSON. So the CURL command looks like this.
curl -H "Authorization: Bearer your-bearer-token" -X POST -d '{"query": "mutation { delete_articles_item(id: 16) { id }}"}' https://directus.example.com/graphql
Advanced GraphQL Usage
Directus combined with GraphQL is very powerful. There are other parameters that can be added to a query to change what data is returned. Below I have combined a few examples such as filters, sort, limit and page.
query {
articles(
filter: { author: first_name: { _eq: "Robert" } } },
sort: ["sort", "-date_created"],
limit: 100,
page: 2
) {
id
title
author {
first_name
}
}
}
What we expect to see is 101 - 200 of articles authored by Robert in descending order of when they where created.
I recommend reading through the Directus documentation for all the possiblities of the filters, such as _eq, _neq, _in, _nin, _between ...
The GraphQL must be wrapped in JSON so the CURL command looks something like this.
curl -H "Authorization: Bearer your-bearer-token" -X POST -d '{"query": "query { articles(filter: { author: first_name: { _eq: "Robert" } } }, sort: ["sort", "-date_created"], limit: 100, page: 2) { id title author { first_name }}}"}' https://directus.example.com/graphql
Conclusion
You can now query, create, update and delete records and perform advanced queries. With these techniques, you can build data-driven applications and websites. If you found this article useful, please subscribe below and I'll share new articles as they become available.