How to perform an API POST Query
REST APIs are a very powerful method for retrieving your data from Directus. In this article, I'll show you how you can perform a POST query using the REST API.
To POST data to the REST API you will need an Authentication Token from Directus with permissions to create items in your collection. Then you can use your favourite server-side scripting language to perform the query.
For this article, I use a free application called Postman to test my API queries and visualise the responses. Let's dive into the first segment - Authentication.
Authentication
Every API is different when it comes to Authentication but in Directus, all you need is a Bearer token. You can create once in the Directus User Directory and generating a token. Make sure the role has permissions to write to the desired collection. This token is required in the authentication header to be accepted by Directus.
In Postman, create a new Collection and open the Authorization tab. Paste your token into the Token field at the bottom.
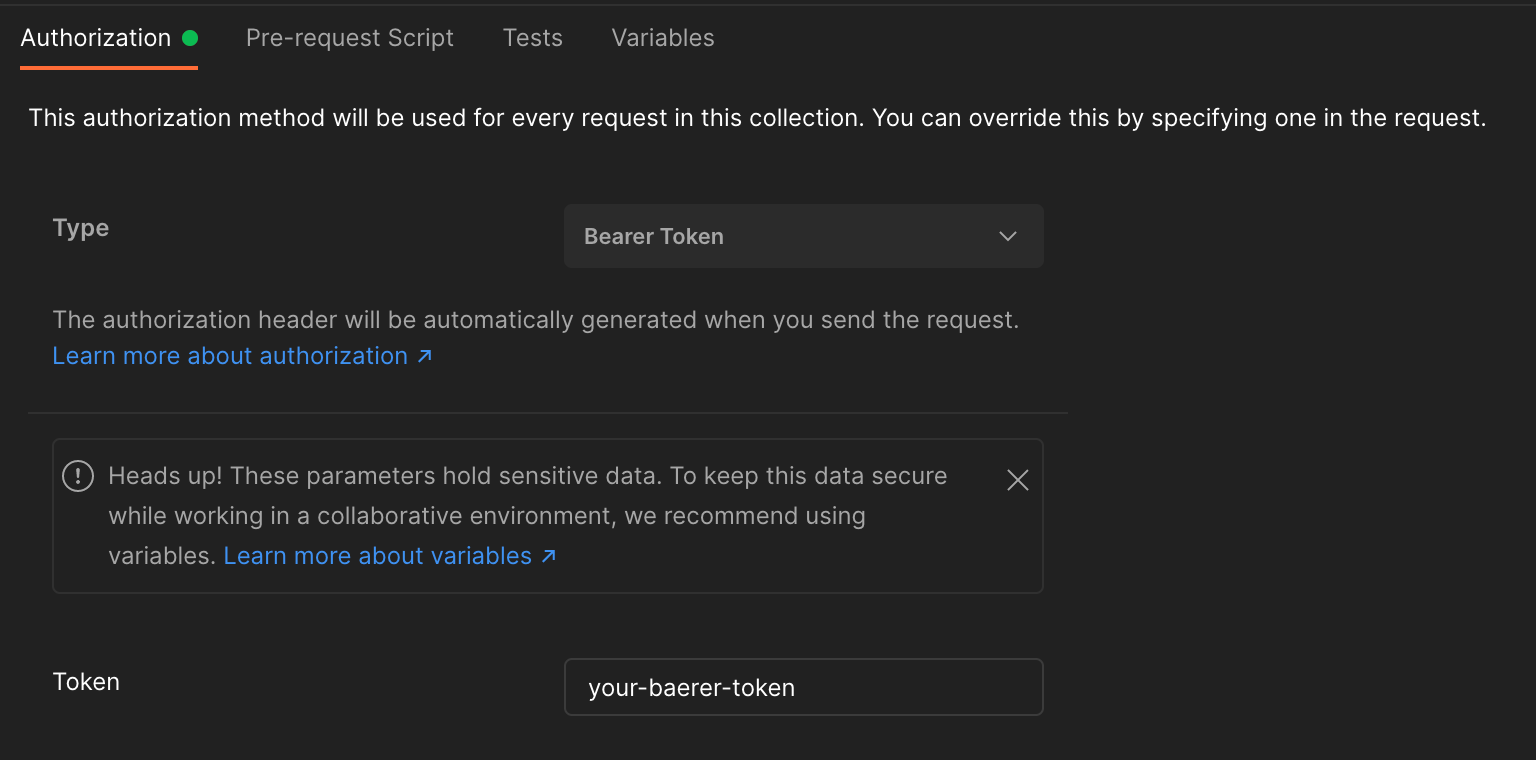
For you application or website, you need to add the token to the authorization header. I'm writing these commands below in raw curl which can be easily translated into your chosen language.
curl -H "Authorization: Bearer your-bearer-token"
Creating a New Record in REST API
To create records using the REST API, use a URL like the one below without any parameters. Note, the URL does not have a trailing slash.
https://directus.example.com/items/articles
Next, we need to POST some data to this URL. It's very important to supply all required fields and honor any field restrictions, such as INT, DATE, BOOLEAN.
In Postman, form data can be posted using either form-data or x-www-form-urlencoded options in the Body tab. Form-data is for simple keys and values. X-www-form-urlencoded is intended for submitting files as well.
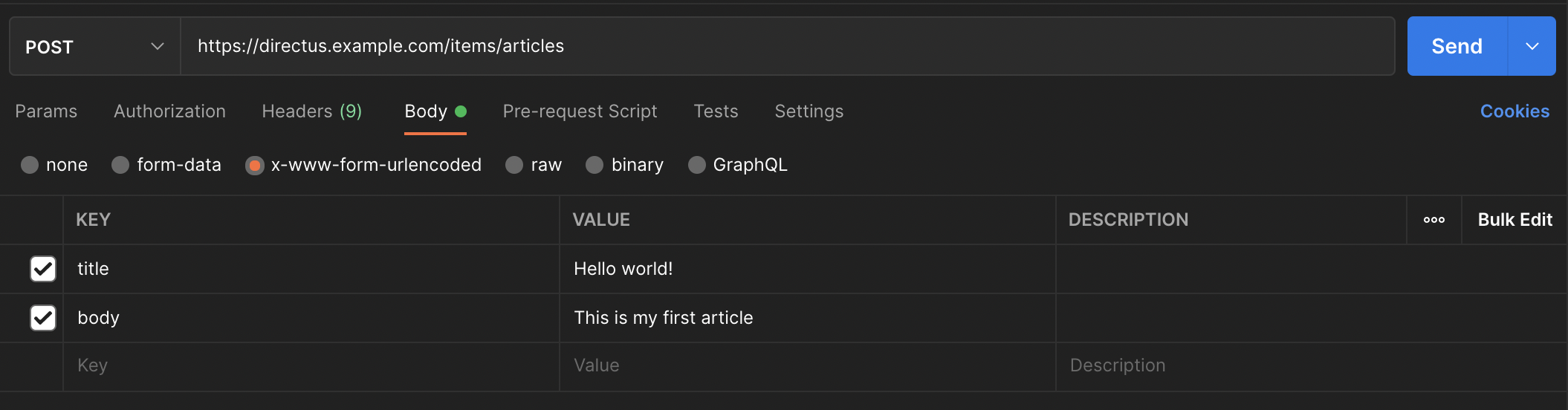
In this example, I'm wanting to send the title and body of my new article into my articles collection. I'm writting this is CURL which can be easily converted to your preferred scripting language. Using CURL, this query looks like this:
curl -H "Authorization: Bearer your-bearer-token" -X POST -F 'title=Hello world!' -F 'body=This is our first article' https://directus.example.com/items/articles
Or if you would prefer x-www-form-urlencoded:
curl -H "Authorization: Bearer your-bearer-token" -X POST -d 'title=Hello world!' -d 'body=This is our first article' https://directus.example.com/items/articles
The JSON response contains the full scope of the record which you can use as confimation.
{
data: {
"id": 16,
"status": "published",
"date_created": "2022-02-05T13:00:00.000Z",
"date_modified": null,
"user_created": "bb6b83ed-aad3-4d6f-be43-1361cd9162cc",
"user_modified": null,
"title": "Hello world!",
"body": "This is my first article",
"author": 1
}
}
Update a Record in REST API
To update an existing record in your collection, you must change the method from POST to PATCH and also supply the ID in the URL. Here is an example:
https://directus.example.com/items/articles/16
PATCH does not accept form-data, instead, you must use raw JSON in the body of the query. In Postman, you can set this up by choosing the PATCH method, then set the body to raw and the syntax to JSON.
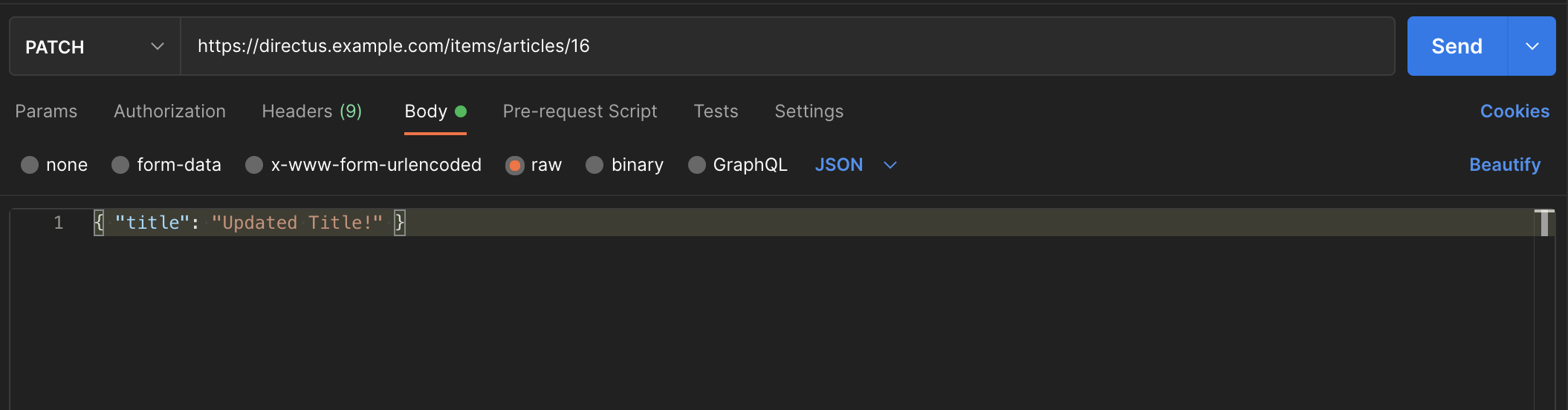
For this example, I'm updating the title of my article to "Updated Title!". Only include the key and value of the fields to update.
Using CURL, the query looks like this:
curl -H "Authorization: Bearer your-bearer-token" -H "Content-type: application/json" -X PATCH -d '{"title": "Hello world!"}' https://directus.example.com/items/articles/16
A few things to note:
- The Content-type header is set to application/json
- The JSON is inside single quotes
- The response will be the same as POST
Further Reading
I find the Directus documenation covers the API very well. Be sure to bookmark the site.
Conclusion
You now have the essential tools to get started with the Directus REST API and the invaluable tool, Postman, for testing the API and understanding the response.